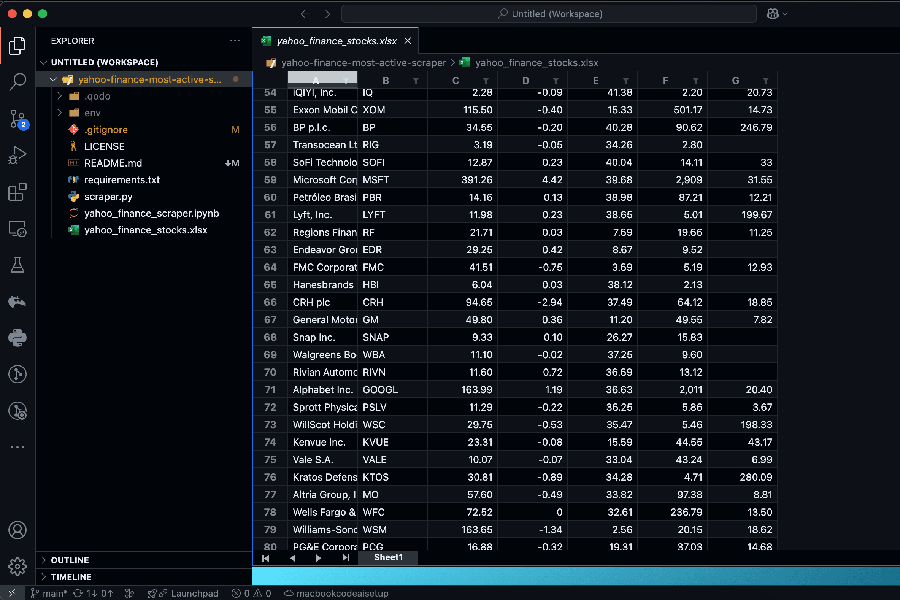
How To Building a Yahoo Finance Most Active Stocks Scraper: A Step-by-Step Guide to Web Scraping with Python
In today’s data-driven world, access to real-time financial data is crucial for investors, analysts, and businesses. However, manually collecting this data can be time-consuming and error-prone. That’s where automation comes in!
In this article, I’ll walk you through how I built a Python-based web scraper to extract the most actively traded stocks data from Yahoo Finance. This tool automates the entire process, saving time and providing structured data for analysis. Whether you’re an investor, data enthusiast, or business owner, this project demonstrates how web scraping can unlock valuable insights from the web.
Why This Project?
The goal of this project was to create a tool that could efficiently scrape and organize financial data, making it easier for users to analyze market trends and make informed decisions.
Target Audience:
-
Investors: Track the most active stocks in real-time.
-
Data Analysts: Use the data for market analysis and visualization.
-
Businesses: Monitor competitors or industry trends.
By automating data collection, this scraper eliminates the need for manual work and ensures accuracy and consistency.
Technologies Used
Here are the key tools and libraries I used to build this scraper:
-
Python: The core programming language for scripting and automation.
-
Selenium: For browser automation and interacting with dynamic web pages.
-
Pandas: For cleaning, organizing, and manipulating the scraped data.
-
OpenPyXL: For saving the data to an Excel file.
-
NumPy: For numerical operations during data cleaning.
1. Setting Up the Environment
Before diving into the code, I set up the environment by installing the required libraries. Here’s how you can do it:
pip install selenium pandas numpy openpyxl
I also downloaded ChromeDriver (to automate the Chrome browser) and added it to my system’s PATH.
2. Accessing Yahoo Finance
Using Selenium, I automated the browser to navigate to Yahoo Finance and locate the "Most Active Stocks" section. Here’s a snippet of the code:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize the WebDriver
driver = webdriver.Chrome()
driver.get("https://finance.yahoo.com/")
# Wait for the page to load
wait = WebDriverWait(driver, 10)
wait.until(EC.presence_of_element_located((By.TAG_NAME, "body")))
This code opens Yahoo Finance and waits for the page to load completely.
3. Extracting and Cleaning Data
Once on the "Most Active Stocks" page, I extracted data such as stock symbols, names, prices, changes, volumes, and market caps. Here’s how I did it:
# Locate the table and extract rows
table = driver.find_element(By.TAG_NAME, "table")
rows = table.find_elements(By.TAG_NAME, "tr")
# Extract data from each row
data = []
for row in rows:
cells = row.find_elements(By.TAG_NAME, "td")
if len(cells) > 0:
stock = {
"symbol": cells[0].text,
"name": cells[1].text,
"price": cells[2].text,
"change": cells[3].text,
"volume": cells[5].text,
"market_cap": cells[7].text,
}
data.append(stock)
After extracting the data, I used Pandas to clean and format it. For example, I removed unnecessary symbols (e.g., +
, M
, B
) and converted text to numerical values.
4. Saving the Data
Finally, I saved the cleaned data to an Excel file using OpenPyXL:
import pandas as pd
# Convert data to a DataFrame
df = pd.DataFrame(data)
# Save to Excel
df.to_excel("most_active_stocks.xlsx", index=False)
The output is a clean, structured Excel file ready for analysis.
This project taught me several valuable lessons:
-
Robust Error Handling: Dynamic web pages can be unpredictable, so it’s important to handle exceptions and edge cases.
-
Data Cleaning: Cleaning and formatting data is a critical step in any data-related project.
-
Automation Saves Time: What used to take hours of manual work can now be done in minutes.
The scraper is highly customizable and can be extended to scrape additional data points or integrate with other tools.
If you’re interested in building a similar tool or need help with web scraping, data analysis, or automation, feel free to reach out! I’d love to discuss how I can help you solve your data challenges.
📩 Let’s connect! Drop me a message or comment below, and let’s start a conversation.
🔗 Check out the project on GitHub: Yahoo Finance Most Active Stocks Scraper
Web scraping is a powerful skill that can unlock valuable insights from the web. Whether you’re an investor, analyst, or business owner, automating data collection can give you a competitive edge. I hope this article inspires you to explore the possibilities of web scraping and automation.
If you found this article helpful, feel free to share it with your network. Let’s spread the knowledge! 🚀